Referencing component children with queries
Components can leverage queries to locate specific elements within their template and potentially access data or functionalities associated with them.
- View Queries:These queries search for elements within the component's view, including direct children and elements further down the DOM tree. They can target components, directives, or even plain DOM elements.
- Content Queries: These queries focus on elements projected into the component's content area using ng-content. This typically involves child components projecting their content into the parent.
- Accessing child component properties or methods:You can retrieve a reference to a child component and then interact with its public properties or methods.
- Manipulating child element DOM:By obtaining a reference to a DOM element, you can directly modify its styles or attributes.
- Reading data from child element injectors:Queries can access specific values provided by child element injectors, offering more control over data retrieval.
View queries
Code Snippet
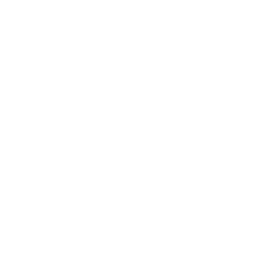
import { Component, ViewChild } from '@angular/core';
@Component({
selector: 'app-ref-view-child',
templateUrl: './ref-view-child.component.html',
styleUrl: './ref-view-child.component.scss'
})
export class RefViewChildComponent {
// Accesing html element using ViewChild. Here test is ref keywork for sample html code shows below
@ViewChild('test') test: any;
ngAfterViewInit() {
console.log(this.test);
}
HTML Code
Code Snippet
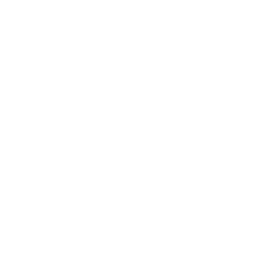
<div #test>
This is example division for ViewChild
</div>
@ViewChildren
For More Details please Visit Its Show Time
Code Snippet
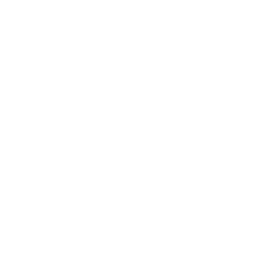
import { Component, ViewChild } from '@angular/core';
@Component({
selector: 'app-ref-view-child',
templateUrl: './ref-view-child.component.html',
styleUrl: './ref-view-child.component.scss'
})
export class RefViewChildComponent {
// Accesing html element using ViewChild. Here test is ref keywork for sample html code shows below
@ViewChildren('test') test: any; it will be QueryList array
ngAfterViewInit() {
this.test.forEach(action => {
console.log(action);
});
}
HTML Code
Code Snippet
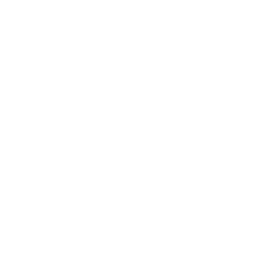
<div #test>
This is example division for ViewChild
</div>
<div #test>
This is example division for ViewChild
</div>
<div #test>
This is example division for ViewChild
</div>
<div #test>
This is example division for ViewChild
</div>
Query locators
Code Snippet
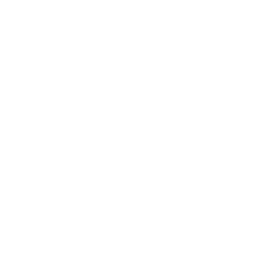
<button #savebtn>Save</button>
HTML Code
Code Snippet
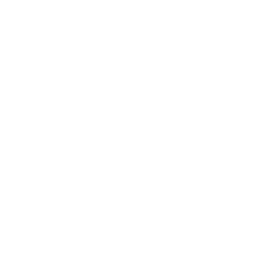
@ViewChild('savebtn') saveButton: ElementRef<HTMLButtonElement>;
Content Children
Conclusion
Leave a Comment
This sample demonstrates the full features of Rich Text Editor that includes all the tools and functionalities.
Comments