Demystifying the Angular Component Lifecycle - Prime Vyapar
Angular components are the building blocks of your web applications...
Understanding the Lifecycle Phases
Imagine a component's life as a journey with distinct stages...
- **Creation:** Angular instantiates the component class...
- **Content Projection:** If the component uses content projection...
- **Initialization:** The `ngOnInit` hook is called, providing...
- **Change Detection:** Angular constantly monitors for changes...
- **Component Rendering:** Based on the component's current state,...
- **Content Checked:** Hooks like `ngAfterContentChecked` and `ngAfterViewChecked`...
- **Changes Detected Throughout:** The change detection cycle can be triggered...
- **Destruction:** When a component is no longer needed (e.g., due to navigation),...
Leveraging Lifecycle Hooks for Powerful Components
By understanding these lifecycle hooks, you gain immense control over your components...
- **
ngOnInit
:** Fetch initial data, set up subscriptions,... - **
ngAfterViewInit
:** Access elements in the component's view... - **
ngOnDestroy
:** Unsubscribe from Observables, remove event listeners,... - **Change Detection Hooks:** Use these for scenarios where you need to react...
Life Cycle Summary
Ref
Phase | Method | Summary |
---|---|---|
Creation | constructor | Standard JavaScript class constructor . Runs when Angular instantiates the component. |
Change Detection | ngOnInit | Runs once after Angular has initialized all the component's inputs. |
ngOnChanges | Runs every time the component's inputs have changed | |
ngDoCheck | Runs every time this component is checked for changes | |
ngAfterViewInit | Runs once after the component's view has been initialized. | |
ngAfterContentInit | Runs once after the component's content has been initialized. | |
ngAfterViewChecked | Runs every time the component's view has been checked for changes. | |
ngAfterContentChecked | Runs every time this component content has been checked for changes.. | |
Rendering | afterNextRender | Runs once the next time that all components have been rendered to the DOM. |
afterRender | Runs every time all components have been rendered to the DOM. | |
Destruction | ngOnDestroy | Runs once before the component is destroyed. |
ngOnInit
Code Snippet
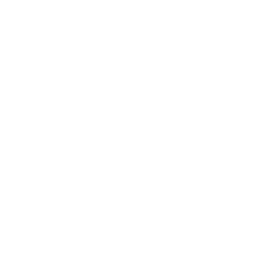
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-my-component',
templateUrl: './my-component.component.html',
styleUrls: ['./my-component.component.css']
})
export class lifeCycle implements OnInit {
name: string = '';
// below method will execute first time when y our compoenent will be l oaded (delay of 1 second)
ngOnInit() {
setTimeout(() => {
this.name = 'Sharad Vishwakarma';
}, 1000);
}
}
ngOnChanges
Code Snippet
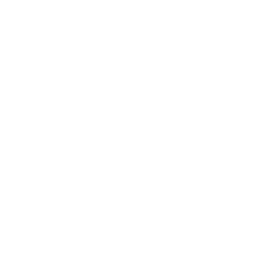
@Component({
selector: 'app-life-cycle',
templateUrl: './life-cycle.component.html',
styleUrl: './life-cycle.component.scss'
})
export class LifeCycleComponent implements OnChanges {
@Input() values =''
ngOnChanges(changes: SimpleChanges): void {
if(changes){
console.log(changes['previousValue']);
console.log(changes['currentValue']);
console.log(changes['firstChange']);
}
}
ngDoCheck
Code Snippet
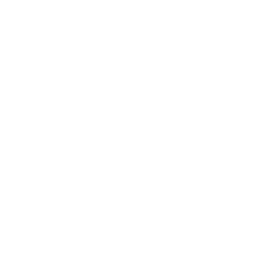
@Component({
selector: 'app-life-cycle',
templateUrl: './life-cycle.component.html',
styleUrl: './life-cycle.component.scss'
})
export class LifeCycleComponent implements OnChanges {
@Input() values =''
ngOnChanges(changes: SimpleChanges): void {
if(changes){
console.log(changes['previousValue']);
console.log(changes['currentValue']);
console.log(changes['firstChange']);
}
}
Conclusion
The Angular component lifecycle offers a powerful mechanism for managing...
**Ready to take your Angular development skills to the next level?**
Explore Angular documentation blog on component lifecycle hooks for in-depth explanations and code : Angular
Leave a Comment
This sample demonstrates the full features of Rich Text Editor that includes all the tools and functionalities.
Comments