Programmatically rendering components - Angular
Using NgComponentOutlet
Code Snippet
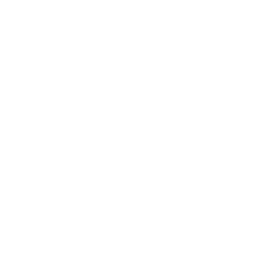
<ng-container *ngComponentOutlet="getCustomComponent()" />
Code Snippet
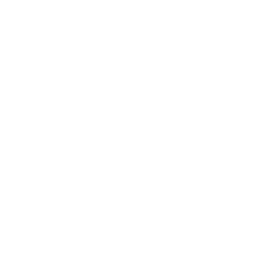
getCustomComponent() {
return this.isAdmin ? AdminBio : StandardBio; //Assuming here we have proper variable/property declaration/component
}
Using ViewContainerRef
Code Snippet
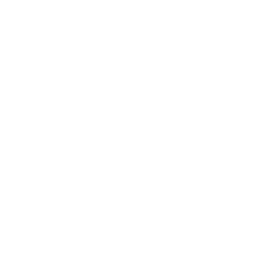
<div>
<ng-container #myContainer></ng-container>
</div>
Code Snippet
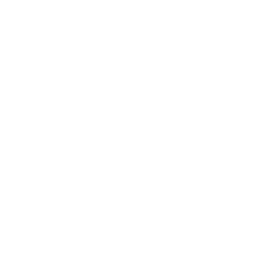
import { Component, ViewChild, ViewContainerRef } from '@angular/core';
@Component({
selector: 'app-my-component',
template:
})
export class MyComponent {
@ViewChild('myContainer', { read: ViewContainerRef })
container: ViewContainerRef;
// Example: Dynamically create and insert a new component
createComponent() {
const component = this.container.createComponent(SomeComponent);
}
}
In this example, the @ng-container> with #myContainer acts as the placeholder. The component class injects ViewContainerRef using @ViewChild and assigns it to the container property. This allows the component to interact with the DOM container and dynamically add components like SomeComponent using createComponent.
- Template Placeholder: In your HTML template, you define a placeholder element (often <ng-container>) to mark the location where you want to dynamically insert components.
- Component Class Interaction: Within your component class, you use @ViewChild to get a reference to this placeholder element and access the associated ViewContainerRef instance.
- Dynamic Component Management: Using the ViewContainerRef methods (e.g., createComponent), you can dynamically create and insert new components into the container at runtime.
Leave a Comment
This sample demonstrates the full features of Rich Text Editor that includes all the tools and functionalities.
Comments