import { Component, Output, EventEmitter } from '@angular/core';
@Component({
selector: 'app-my-counter',
template:
Current Count: {{ count }}
})
export class MyCounterComponent {
count = 0;
@Output() countChanged = new EventEmitter();
increment() {
this.count++;
this.countChanged.emit(this.count);
}
}
Supercharge Your Angular Apps: Custom Events with Outputs
Angular, a powerful framework for building dynamic web applications, provides a robust mechanism for component communication: custom events with outputs. This approach fosters modularity, reusability, and cleaner code, keeping your Angular projects well-organized and interactive.
Understanding Custom Events and Outputs
In Angular, custom events allow components to communicate by emitting and listening to events they define themselves. These events are not part of the standard DOM event model, but they offer more control and flexibility for building custom functionalities within your application.
The Power of @Output
The `@Output` decorator is the key to creating custom events in Angular components. It marks a property within your component class as an output, allowing other components to listen for it.
Code Snippet
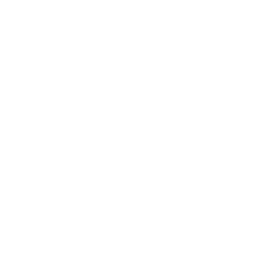
In this example, the `MyCounterComponent` defines a `countChanged` property decorated with `@Output`. It's an instance of `EventEmitter`, a class that helps manage event emission in Angular.
Listening with Event Binding
Once a component defines a custom event, other components can listen for it using event binding syntax in the template.
Code Snippet
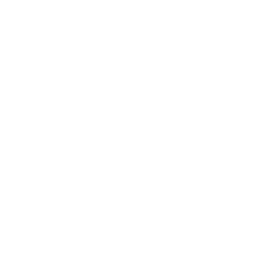
<app-my-counter (countChanged)="onCountChange($event)">></app-my-counter>
// In the parent component class
onCountChange(newCount: number) {
console.log('Count changed to:', newCount);
}
Here, the parent component listens for the `countChanged` event emitted by `MyCounterComponent`. When the event is fired, the `onCountChange` method is called, receiving the new count value.
Benefits of Custom Events with Outputs
Using custom events with outputs in Angular offers several advantages:
- **Modular Communication:** Components can interact without tight coupling, promoting cleaner code and easier maintenance.
- **Reusability:** Components become more reusable as they can emit events for common functionalities.
- **Flexibility:** You can define events with specific data types for targeted communication.
In Conclusion
By leveraging custom events with outputs, you can create a more modular, interactive, and well-structured Angular application. This approach promotes cleaner component interactions and simplifies communication between different parts of your application.
Remember
While custom events are powerful, use them strategically. Consider built-in Angular features and events whenever possible to maintain code clarity. However, for unique functionalities and complex interactions, custom events with outputs provide a valuable tool in your Angular development arsenal.
Leave a Comment
This sample demonstrates the full features of Rich Text Editor that includes all the tools and functionalities.
Comments